React.js and Supabase Masterclass – Build Full-Stack Apps from Scratch
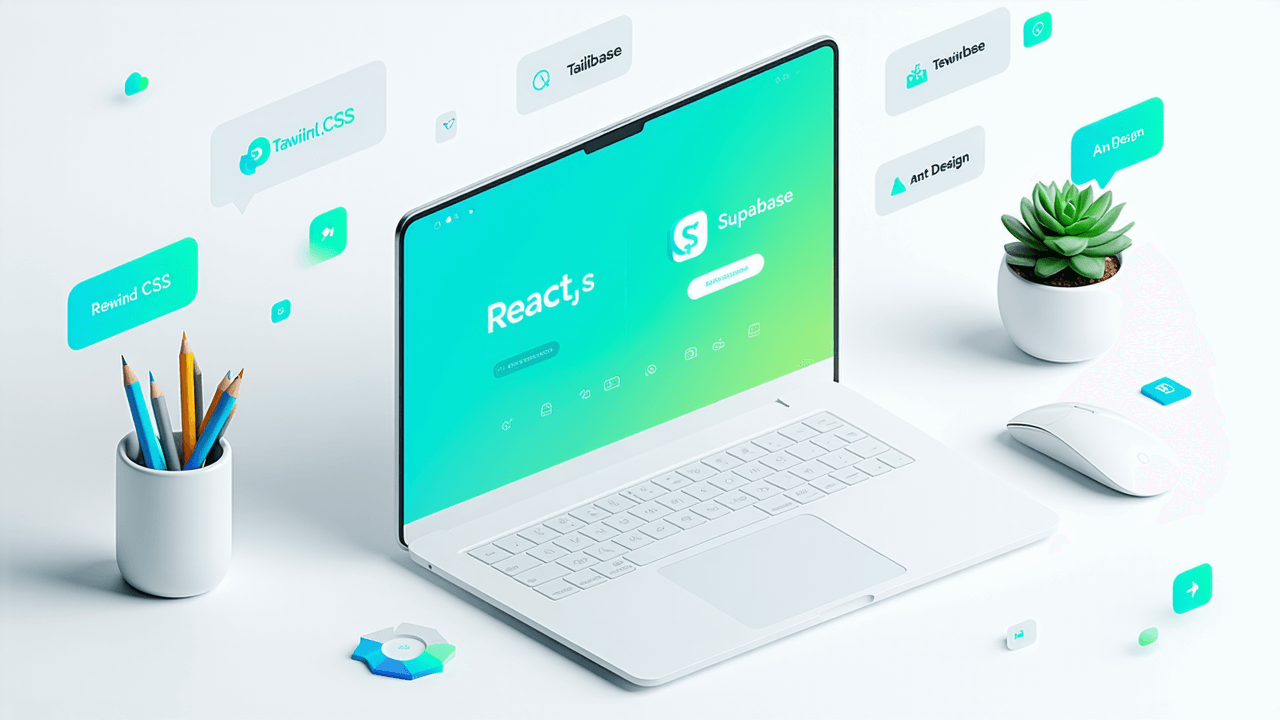
React.js and Supabase Tutorial – Build Full-Stack Apps with Tailwind CSS & Ant Design
Introduction to Full-Stack Development with React and Supabase
Welcome to your complete React.js and Supabase tutorial!
In this hands-on guide, you’ll learn how to:
- Build a full-stack web application using React and Supabase
- Add styling with Tailwind CSS
- Use ready-made components with Ant Design
- Set up full user authentication (sign up, login, logout)
- Build a complete CRUD (Create, Read, Update, Delete) system
- Upload and display images with Supabase Storage
- Deploy your application online using Vercel
By the end, you’ll have a solid, production-ready full-stack app ready for the real world!
Prerequisites
Before we get our hands dirty, make sure you have these essentials:
- ✅ Node.js installed (Download here)
- ✅ A code editor like Visual Studio Code
- ✅ A free Supabase account (Sign up here)
- ✅ Basic familiarity with JavaScript, HTML, and CSS
If you’re comfortable running a few terminal commands and writing basic JavaScript, you’re good to go!
Setting Up Your React Project with Vite
Let’s kick things off by creating a brand-new React project using Vite, which makes development blazing fast.
Open your terminal and run:
npm create vite@latest react-supabase-app -- --template react
cd react-supabase-app
npm install
npm run dev
You should now be able to visit
http://localhost:5173
and see your React app live!
Adding Tailwind CSS for Quick Styling
Next, let’s bring in Tailwind CSS to style our app effortlessly.
Install Tailwind and its dependencies:
npm install -D tailwindcss postcss autoprefixer npx tailwindcss init -p
Then, update your tailwind.config.js :
module.exports = {
content: ["./index.html", "./src/**/*.{js,ts,jsx,tsx}"],
theme: {
extend: {},
},
plugins: [],
}
In your src/index.css, add the Tailwind directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
Done! Tailwind is now fully integrated.
Integrating Ant Design Components
Time to make your UI pop with Ant Design!
Install it with:
npm install antd
Import AntD’s reset CSS in main.jsx :
import 'antd/dist/reset.css';
Now, use a ready-made Ant Design button in App.jsx :
import { Button } from 'antd';
function App() {
return (
<div className="p-4">
<Button type="primary">Get Started</Button>
</div>
);
}
export default App;
You’ll see a beautiful blue button ready to go!
Creating Your Supabase Project
Go to Supabase Dashboard, click New Project, and:
- Set a Project Name
- Choose a Password (store it safely)
- Select the region closest to you
Supabase will spin up your database in a few seconds.
Setting Up the Supabase Client in React
Let’s connect React to Supabase.
Install the client library:
npm install @supabase/supabase-js
Create a file called src/supabaseClient.js:
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = 'https://your-project-id.supabase.co';
const supabaseKey = 'your-anon-key';
export const supabase = createClient(supabaseUrl, supabaseKey);
Replace the placeholders with your actual project URL and anon key from the Supabase settings.
Implementing User Authentication with Supabase
Handling authentication is super simple with Supabase. Let’s build Sign Up, Sign In, and Sign Out flows inside your React app.
1. Sign Up Users
First, let’s create a sign-up function in your App.jsx:
import { supabase } from './supabaseClient';
import { useState } from 'react';
import { Button, Input, message } from 'antd';
export default function App() {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const handleSignUp = async () => {
const { error } = await supabase.auth.signUp({ email, password });
if (error) {
message.error(error.message);
} else {
message.success('Sign-up successful! Check your email.');
}
};
return (
<div className="p-8 max-w-md mx-auto">
<Input
placeholder="Email"
onChange={(e) => setEmail(e.target.value)}
className="mb-4"
/>
<Input.Password
placeholder="Password"
onChange={(e) => setPassword(e.target.value)}
className="mb-4"
/>
<Button type="primary" onClick={handleSignUp}>Sign Up</Button>
</div>
);
}
2. Sign In Users
Let’s add a simple login function:
const handleSignIn = async () => {
const { error } = await supabase.auth.signInWithPassword({ email, password });
if (error) {
message.error(error.message);
} else {
message.success('Signed in successfully!');
}
};
Attach it to another button if you want:
<Button type="default" onClick={handleSignIn}>Sign In</Button>
3. Sign Out Users
Logging out is a breeze:
const handleSignOut = async () => {
const { error } = await supabase.auth.signOut();
if (error) {
message.error(error.message);
} else {
message.success('Signed out successfully!');
}
};
Building a Full CRUD Application
Let’s make a simple Task Manager App that supports Create, Read, Update, and Delete operations!
1. Set Up Your Database Table
Go to the Supabase Dashboard → Table Editor → New Table:
- Table name: tasks
- Columns:
- id (UUID, Primary Key, Default Value: gen_random_uuid())
- user_id (UUID)
- title (Text)
- is_complete (Boolean, Default: false)
- created_at (Timestamp)
Make sure "user_id" is foreign keyed to "auth.users"!
2. Create New Tasks
Add a function in your app:
const addTask = async (title) => {
const { data, error } = await supabase
.from('tasks')
.insert([{ title, user_id: supabase.auth.user().id }]);
if (error) console.error(error);
};
3. Read Tasks
Display all the tasks belonging to the logged-in user:
const fetchTasks = async () => {
const { data, error } = await supabase
.from('tasks')
.select('*')
.eq('user_id', supabase.auth.user().id);
if (error) console.error(error);
setTasks(data);
};
4. Update Tasks
Toggle a task’s completion:
const toggleTask = async (task) => {
const { data, error } = await supabase
.from('tasks')
.update({ is_complete: !task.is_complete })
.eq('id', task.id);
if (error) console.error(error);
};
5. Delete Tasks
Remove a task:
const deleteTask = async (id) => {
const { error } = await supabase
.from('tasks')
.delete()
.eq('id', id);
if (error) console.error(error);
};
Handling Supabase Storage for File Uploads
Want users to upload profile pictures or attachments?
Here’s how to handle file uploads with Supabase Storage!
1. Create a Storage Bucket
In Supabase Dashboard → Storage → Create Bucket (e.g., avatars).
2. Upload a File
const uploadFile = async (file) => {
const { data, error } = await supabase.storage
.from('avatars')
.upload(`public/${file.name}`, file);
if (error) console.error(error);
else console.log('File uploaded:', data);
};
Let users select a file:
<input type="file" onChange={(e) => uploadFile(e.target.files[0])} />
Creating a Responsive UI with Ant Design
Make your app beautiful and responsive!
Example: Display tasks in a styled Card:
import { Card, Button } from 'antd';
<Card title={task.title} extra={<Button onClick={() => deleteTask(task.id)}>Delete</Button>}>
<p>Status: {task.is_complete ? "Completed" : "Pending"}</p>
</Card>
You can even use Ant Design Tables, Forms, Modal dialogs, and more!
Managing Global State for Auth and Data
While a simple app can use local state (useState), bigger apps benefit from global state management.
Options:
- ✅ React Context API (good for auth tokens)
- ✅ Redux Toolkit (good for complex apps)
Example Context for Auth:
import { createContext, useContext, useState } from 'react';
const AuthContext = createContext();
export function AuthProvider({ children }) {
const [user, setUser] = useState(null);
return (
<AuthContext.Provider value={{ user, setUser }}>
{children}
</AuthContext.Provider>
);
}
export const useAuth = () => useContext(AuthContext);
Error Handling and Validation
Use AntD's message.error() for user-friendly errors!
Always check:
- Empty form inputs
- Invalid email formats
- API errors (like 400/401 Unauthorized)
This ensures your app doesn’t crash and users get clear feedback.
Deploying Your Full-Stack App on Vercel
Let’s go live! 🌐
Steps:
- Push your project to GitHub.
- Create a Vercel account (Sign up).
- Click New Project → Import GitHub Repo.
- Set the environment variables:
- VITE_SUPABASE_URL
- VITE_SUPABASE_ANON_KEY
- Click Deploy!
Within minutes, your full-stack React Supabase app will be live!
Final Thoughts and Next Steps
Congrats—you’ve built and deployed a full-stack app with:
- ✅ React
- ✅ Supabase (Database + Auth + Storage)
- ✅ Tailwind CSS
- ✅ Ant Design
What’s next?
- Add Role-Based Access Control (RBAC)
- Implement Real-Time Sync (Supabase supports it!)
- Explore Serverless Functions on Vercel
FAQs About React.js, Supabase, and Full-Stack Development
1. Is Supabase free to use?
Yes, Supabase offers a generous free tier. Perfect for personal and small projects!
2. Can I use Supabase with Next.js instead of React?
Absolutely. Supabase works great with Next.js too.
3. Is Tailwind CSS better than plain CSS?
Tailwind offers faster styling, but it's personal preference. Many developers find it much quicker.
4. Do I need Redux for small projects?
Nope! useState and useContext are enough for small to mid-sized apps.
5. How secure is Supabase Authentication?
Supabase uses industry-standard practices like JWT tokens. It's secure for most applications!
6. Can I deploy Supabase itself?
Supabase hosts your backend for you. No need for a separate deployment!
🎉 You Did It!
You’ve now built a real-world, full-stack app using cutting-edge tools—and it’s deployed for the world to see.
🚀 Bonus Section: Real-Time Data Sync and Advanced Filtering in Supabase
Real-Time Data Sync with Supabase Subscriptions
Want your app to automatically update when new tasks are created, updated, or deleted—without manually refreshing the page?
Supabase offers real-time subscriptions right out of the box. Here's how to set it up:
1. Subscribe to Database Changes
Inside your App.jsx or a custom hook, you can listen for changes like this:
import { useEffect } from 'react';
import { supabase } from './supabaseClient';
export default function useRealtimeTasks(setTasks) {
useEffect(() => {
const subscription = supabase
.channel('public:tasks')
.on(
'postgres_changes',
{ event: '*', schema: 'public', table: 'tasks' },
(payload) => {
console.log('Change received!', payload);
setTasks((prevTasks) => {
switch (payload.eventType) {
case 'INSERT':
return [...prevTasks, payload.new];
case 'UPDATE':
return prevTasks.map(task =>
task.id === payload.new.id ? payload.new : task
);
case 'DELETE':
return prevTasks.filter(task => task.id !== payload.old.id);
default:
return prevTasks;
}
});
}
)
.subscribe();
return () => {
supabase.removeChannel(subscription);
};
}, [setTasks]);
}
✅ This ensures that whenever a task is added, updated, or deleted in the database, your UI updates automatically!
Advanced Filtering and Searching with Supabase Queries
Want to build a search bar or sort tasks? Supabase's flexible queries make it easy.
1. Fetch Only Completed Tasks
const fetchCompletedTasks = async () => {
const { data, error } = await supabase
.from('tasks')
.select('*')
.eq('is_complete', true)
.order('created_at', { ascending: false });
if (error) console.error(error);
else setTasks(data);
};
2. Search Tasks by Title
Suppose you want users to find tasks by keyword:
const searchTasks = async (keyword) => {
const { data, error } = await supabase
.from('tasks')
.select('*')
.ilike('title', `%${keyword}%`);
if (error) console.error(error);
else setTasks(data);
};
- .ilike performs a case-insensitive search (like SQL's ILIKE).
- %keyword% matches anywhere inside the title.
✅ Combine this with a simple <Input.Search /> component from Ant Design, and you’ve got a live search feature!
Pro Tip: Paginate Large Data Sets
For apps with lots of data, fetch limited results:
const fetchPaginatedTasks = async (page = 1) => {
const pageSize = 10;
const { data, error } = await supabase
.from('tasks')
.select('*')
.range((page - 1) * pageSize, page * pageSize - 1)
.order('created_at', { ascending: false });
if (error) console.error(error);
else setTasks(data);
};
This keeps your app fast and efficient as your database grows!
🎯 Bonus Section Summary
Thanks to Supabase's powerful features, you can easily:
- ✅ Build real-time apps without needing WebSocket servers.
- ✅ Create dynamic search and advanced filters.
- ✅ Handle large datasets with pagination.
All inside your React.js app—with very little backend work!
📢 Final Wrap-Up
At this point, you haven't just built an app—you've mastered:
- Full-stack development with React.js and Supabase
- Responsive styling using Tailwind CSS and Ant Design
- User authentication, CRUD operations, storage, real-time sync, advanced searching, and more
- Deploying your app to the world with Vercel
🚀 You're officially ready to build your own SaaS projects, client apps, or even launch startups!
Related Articles
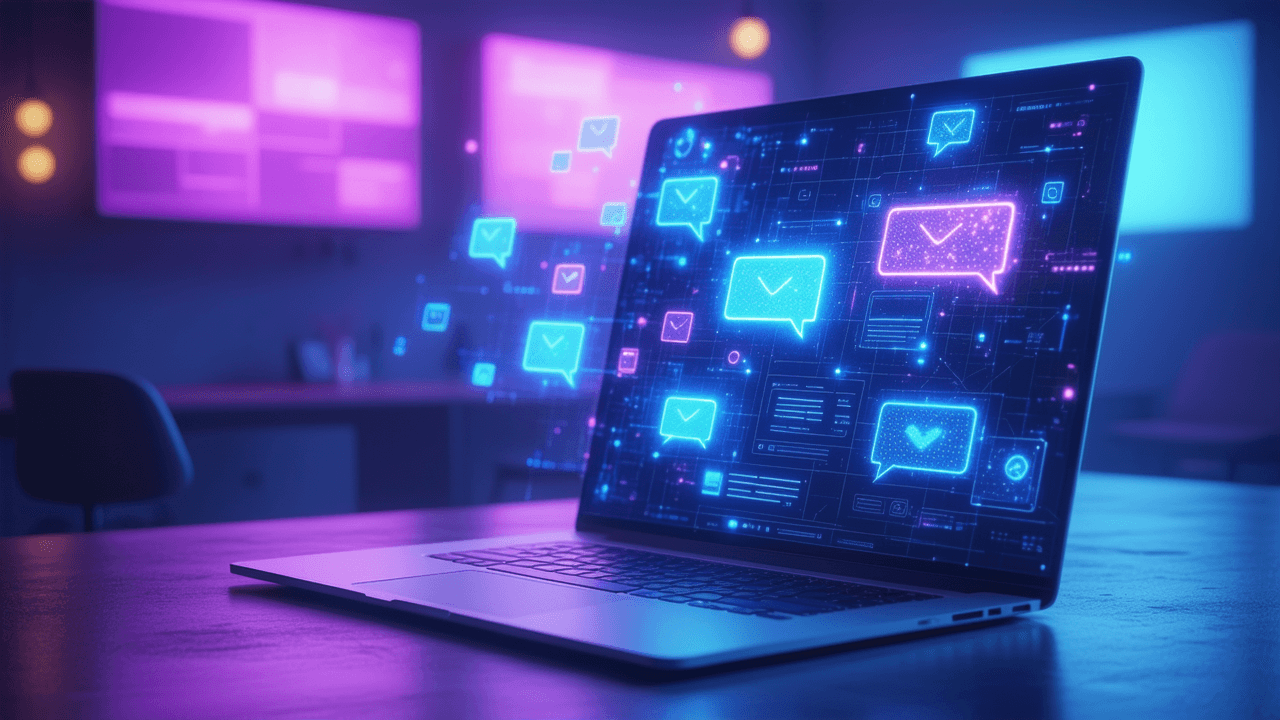
Building AI-Powered Chatbots with LangChain and OpenAI
Learn how to create intelligent conversational interfaces using LangChain and OpenAI's GPT models. A step-by-step guide with code examples.
Read More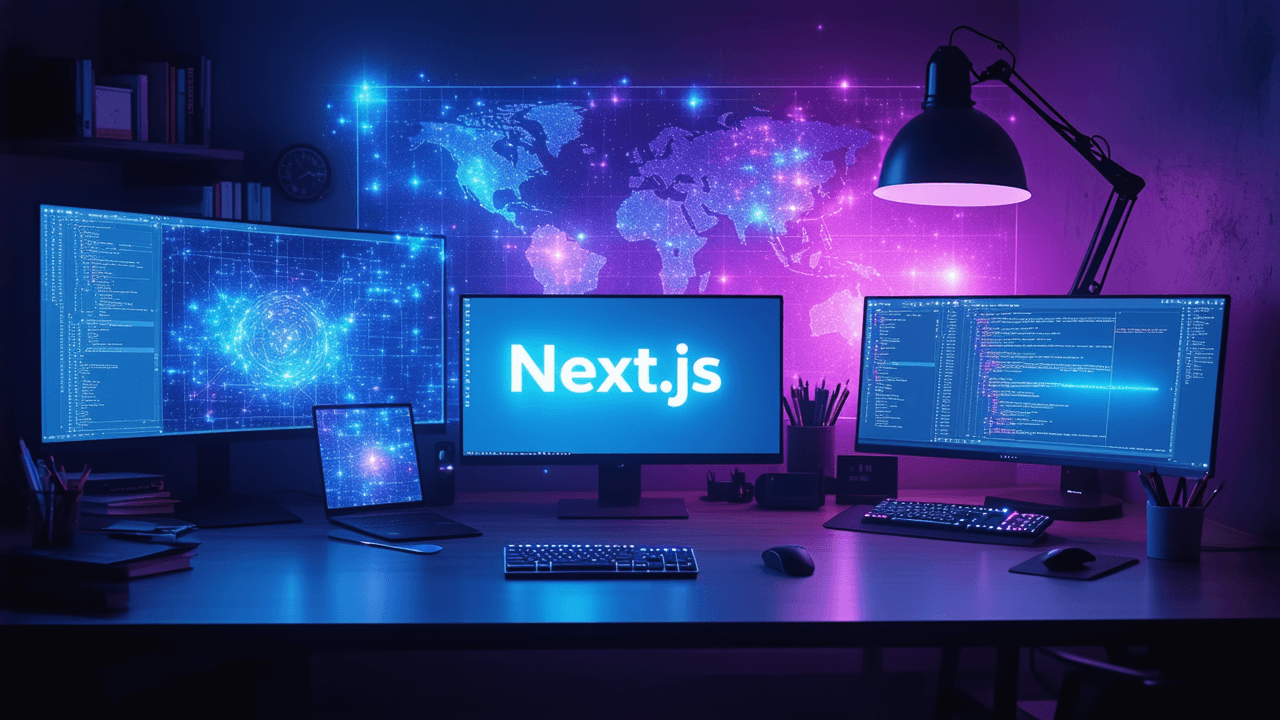
Next.js 14 Features That Will Change Your Development Workflow
Explore the latest features in Next.js 14 and how they can improve your development experience and application performance.
Read More