5 Mind-Blowing AI Apps You Can Build with Linked Lists – #3 Will Shock You!
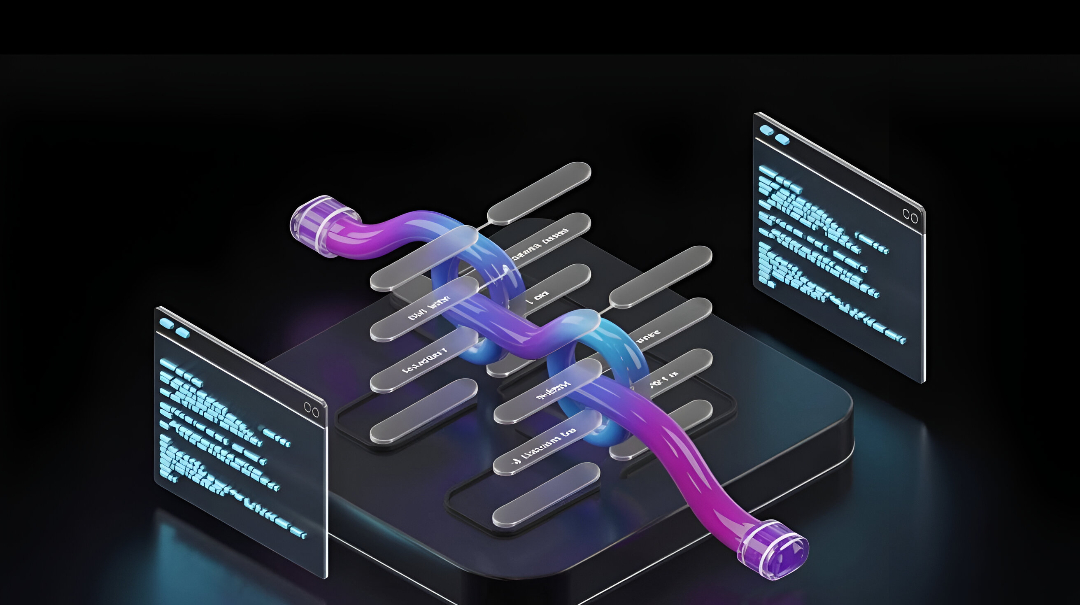
When most people think about artificial intelligence (AI), their minds jump to deep learning, neural networks, or futuristic robots. But here’s the truth: even the most groundbreaking AI systems rely heavily on classic data structures like linked lists. Surprised? You’re not alone.
As an AI Data Structures expert, I can tell you that mastering fundamental data structures is crucial to building scalable, efficient AI applications. In this post, we’re going to show you 5 jaw-dropping AI apps you can build with linked lists. Whether you’re a beginner looking for exciting AI project ideas, or a developer wanting to understand the real-world linked list applications, you’re in the right place.
So buckle up, because #3 on our list might just change how you see data structures in AI forever.
1. AI-Powered Chat Queue Manager
How It Works
AI chatbots are everywhere, from customer service to virtual assistants. But what happens when multiple user requests flood in at once? That’s where a linked list-based queue system becomes invaluable.
Why Use Linked Lists?
Linked lists provide O(1) time complexity for insertion and deletion at both ends (especially in doubly linked lists). This makes them ideal for dynamic environments where user interactions are unpredictable.
Enhanced Implementation (Python Pseudocode)
type ChatRequest = {
'user_id': int,
'timestamp': datetime,
'query': str
}
class Node:
def __init__(self, data: ChatRequest):
self.data = data
self.next = None
class ChatQueue:
def __init__(self):
self.head = None
self.tail = None
def enqueue(self, request):
new_node = Node(request)
if self.tail:
self.tail.next = new_node
self.tail = new_node
if not self.head:
self.head = new_node
def dequeue(self):
if not self.head:
return None
request = self.head.data
self.head = self.head.next
if not self.head:
self.tail = None
return request
AI Layer
Integrate NLP models like BERT to prioritize or tag queries based on sentiment, urgency, or intent. This adds intelligent triage to your queue.
2. Smart Task Scheduler for AI Agents
How It Works
AI agents often juggle multiple tasks. Whether it's a robot cleaning a room or a virtual assistant managing calendar events, efficient task handling is vital for performance and reliability.
Why Use Linked Lists?
Linked lists allow insertion of high-priority tasks in the middle of the list without restructuring, which is computationally expensive with arrays.
Enhanced Implementation (Python Pseudocode)
class Task:
def __init__(self, name, priority):
self.name = name
self.priority = priority
self.next = None
class TaskScheduler:
def __init__(self):
self.head = None
def add_task(self, task):
if not self.head or task.priority > self.head.priority:
task.next = self.head
self.head = task
else:
current = self.head
while current.next and current.next.priority >= task.priority:
current = current.next
task.next = current.next
current.next = task
AI Layer
Use reinforcement learning or decision trees to dynamically update task priorities based on real-time feedback and outcomes.
3. Real-Time Emotion Tracking App
How It Works
This innovative app uses a webcam and facial recognition AI to detect and log emotional states in real time. It's useful for health monitoring, virtual interviews, or adaptive learning environments.
Why Use Linked Lists?
Emotion data is sequential and constantly updated, making singly linked lists ideal for storing a time-ordered stream of emotions efficiently.
Enhanced Implementation (Python Pseudocode)
class EmotionNode:
def __init__(self, timestamp, emotion):
self.timestamp = timestamp
self.emotion = emotion
self.next = None
class EmotionTracker:
def __init__(self):
self.head = None
def add_emotion(self, timestamp, emotion):
new_node = EmotionNode(timestamp, emotion)
new_node.next = self.head
self.head = new_node
def get_recent_emotions(self, limit=5):
current = self.head
count = 0
emotions = []
while current and count < limit:
emotions.append((current.timestamp, current.emotion))
current = current.next
count += 1
return emotions
AI Layer
Integrate facial emotion recognition using CNNs or deep facial landmark models. Classify emotions (e.g., happy, sad, surprised) and analyze temporal patterns using the linked list.
4. Adaptive Learning Path Generator
How It Works
This app generates custom learning paths for users based on progress, learning style, and interaction. It's especially effective in personalized education platforms.
Why Use Linked Lists?
Each lesson (node) can point to the next appropriate lesson. As the user progresses or needs to backtrack, nodes can be rearranged with minimal overhead.
Enhanced Implementation (Python Pseudocode)
class LessonNode:
def __init__(self, topic):
self.topic = topic
self.next = None
class LearningPath:
def __init__(self):
self.head = None
def add_lesson(self, topic):
new_node = LessonNode(topic)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def complete_lesson(self):
if self.head:
self.head = self.head.next
AI Layer
Use supervised learning models to track student performance and predict the next optimal lesson, adjusting the path accordingly.
5. Dynamic Memory Assistant
How It Works
Inspired by how the human brain works, this app mimics short-term and long-term memory using a linked list-based memory store. It can assist users by recalling recent tasks, notes, or contextual reminders.
Why Use Linked Lists?
Linked lists support dynamic memory modeling. New memories (nodes) are added at the head, and older, less-relevant ones can be forgotten or moved to secondary storage.
Enhanced Implementation (Python Pseudocode)
class MemoryNode:
def __init__(self, content):
self.content = content
self.next = None
class MemoryAssistant:
def __init__(self):
self.head = None
def remember(self, content):
node = MemoryNode(content)
node.next = self.head
self.head = node
def forget_oldest(self):
if not self.head:
return
current = self.head
prev = None
while current.next:
prev = current
current = current.next
if prev:
prev.next = None
else:
self.head = None
AI Layer
Use semantic embeddings (e.g., via transformers) to categorize and retrieve relevant memories. This allows contextual reminders and memory pruning.
FAQs About Linked Lists in AI
What are linked lists in AI?
Linked lists are dynamic data structures made up of nodes that hold data and point to the next node. In AI, they are commonly used for building queues, memory buffers, and sequential models.
Why are linked lists better than arrays in some AI apps?
Arrays are static in size and require expensive operations to insert/delete elements. Linked lists adapt quickly to changes, which is key in real-time AI systems.
Are linked lists used in machine learning?
Absolutely. While most libraries abstract them, data pipelines, replay buffers in reinforcement learning, and preprocessing queues often use linked lists.
What are some beginner AI coding projects using linked lists?
- Chatbot queue manager
- Personalized study assistant
- Emotion recognition logger
- Task scheduler for home automation
- AI-powered journaling assistant
Can I combine linked lists with other data structures in AI?
Yes, and you should! Combining linked lists with hash tables, trees, and graphs gives you powerful hybrid data models that improve performance and scalability.
Ready to Build More?
Linked lists are more than textbook material – they’re the foundation of real, powerful AI apps. From chatbots to cognitive systems, linked lists empower flexibility and performance. If you’re excited about what you can build, check out our tutorials on AI data structures, explore our GitHub repo, or join our coding bootcamp for beginner AI coding projects.
Stay curious, keep building, and don’t underestimate the power of a well-placed linked list in your AI stack.
Related Articles
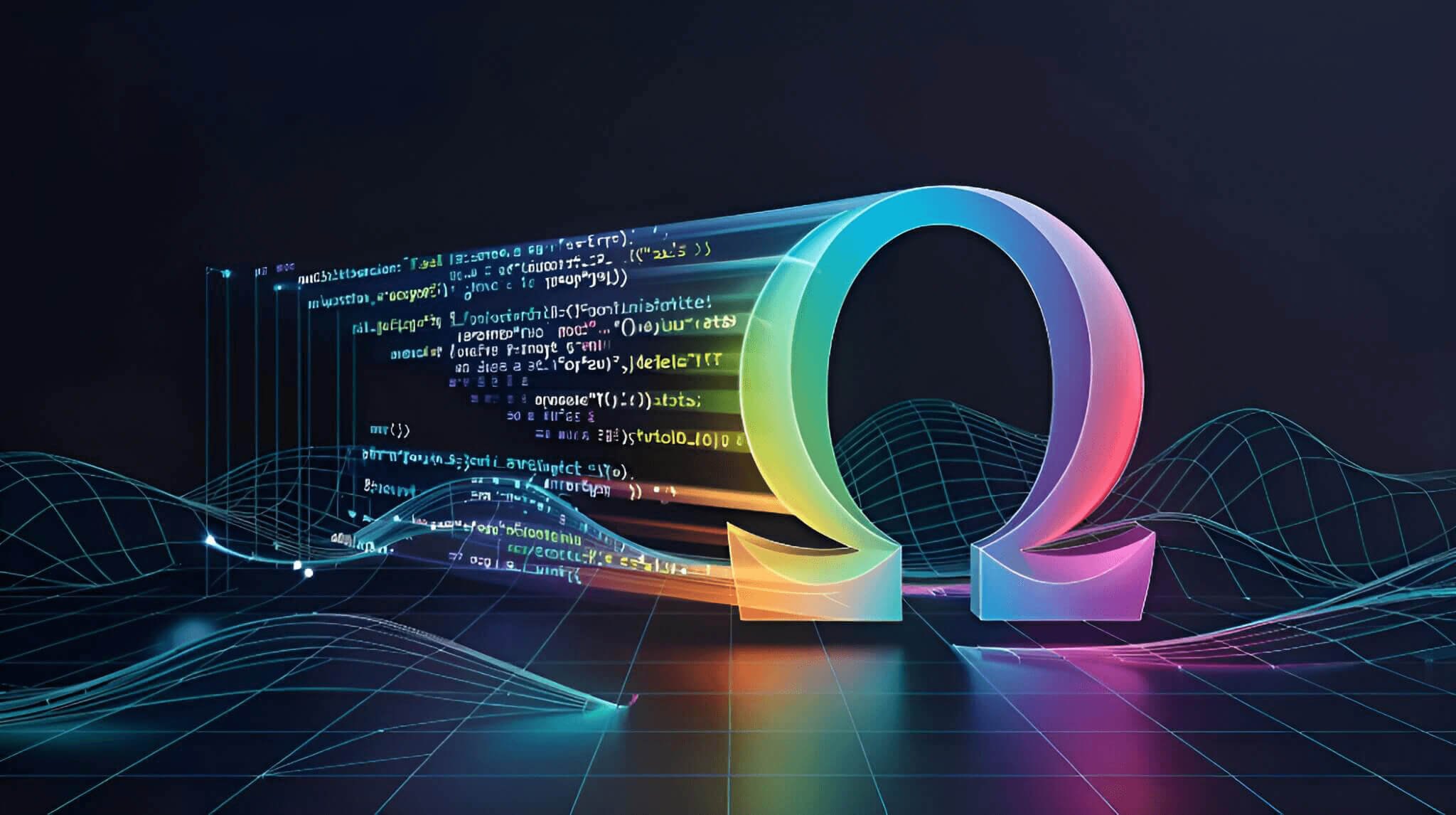
Big Omega, Asymptotic Analysis & Algorithm Complexity in JavaScript
Understand Big Omega notation, algorithm complexity, and asymptotic analysis with clear JavaScript examples, visuals, and embedded sandboxes.
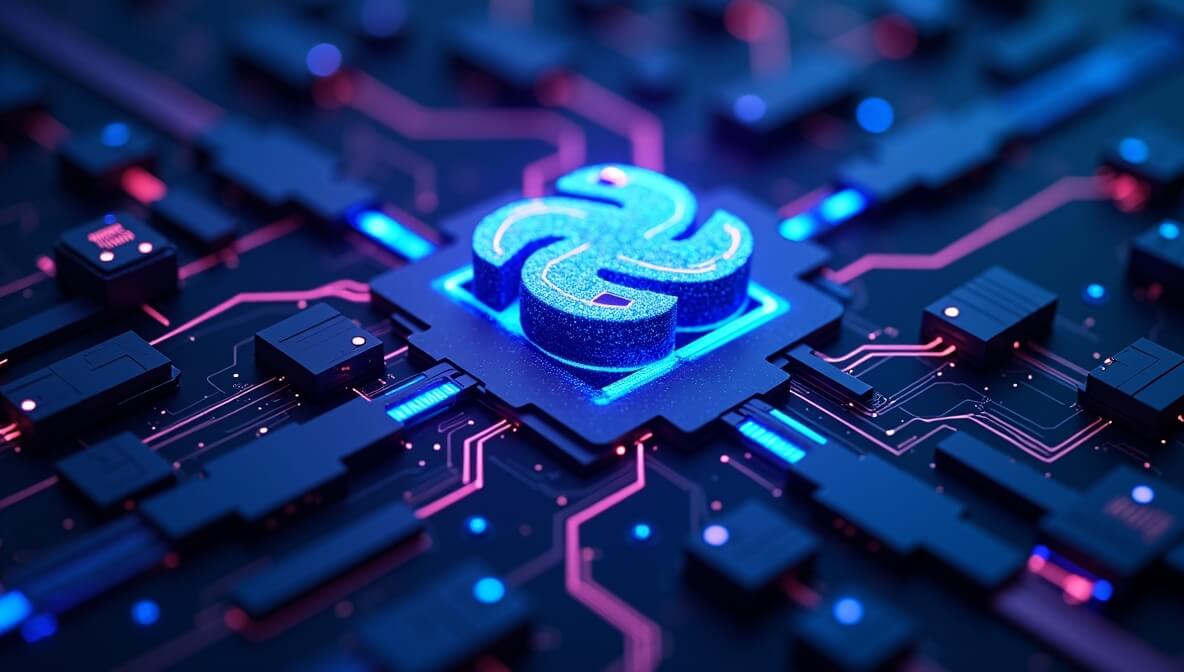
How to Design and Scale AI-Driven APIs Using Python
Discover how to build scalable, cost-efficient AI-driven APIs with Python. Learn best practices, architecture design, bottleneck handling, and multi-model strategies.