Big Omega, Asymptotic Analysis & Algorithm Complexity in JavaScript
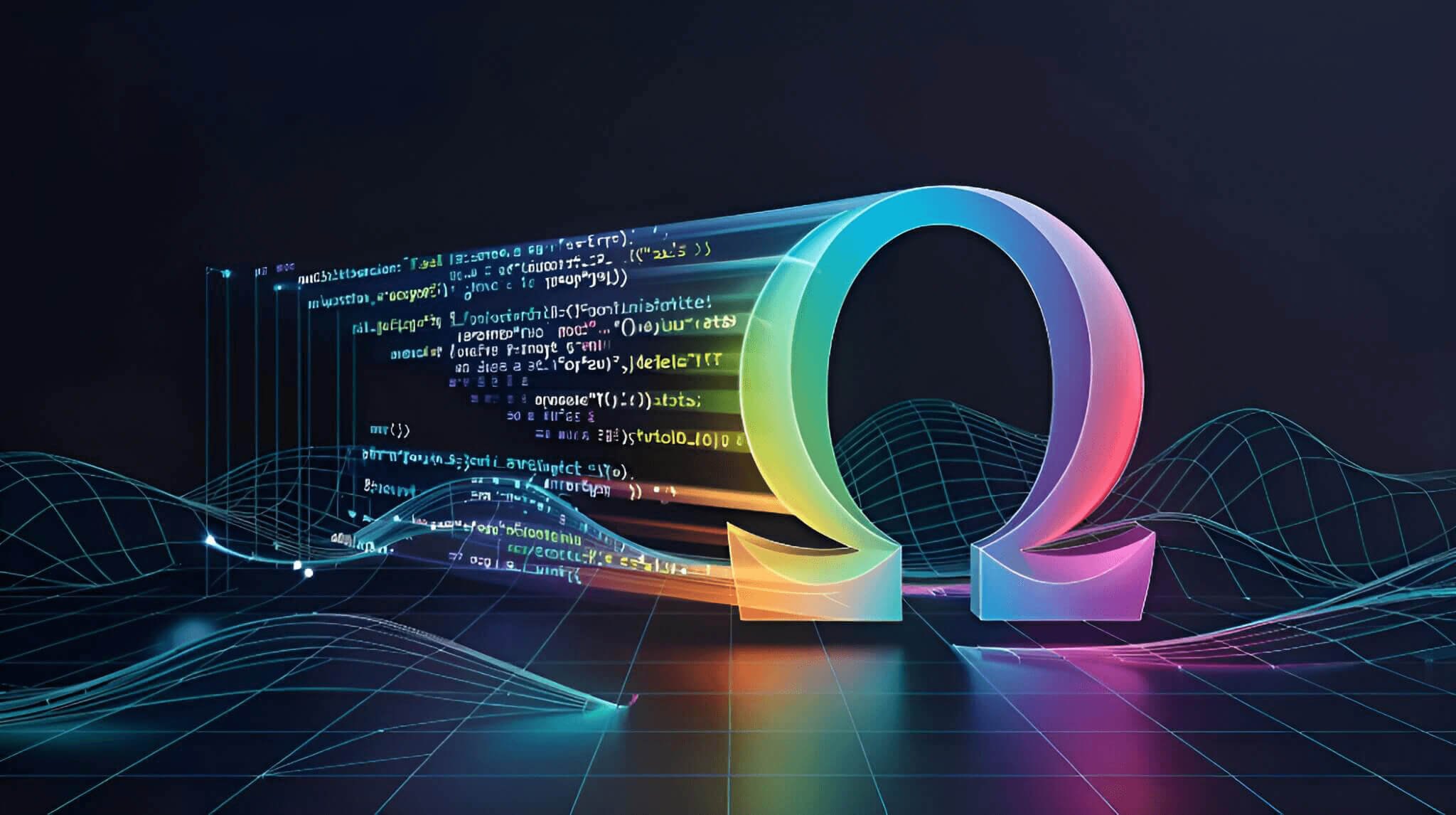
🧠 Introduction: Why Algorithm Analysis Matters
In the fast-evolving world of software development, writing code that merely works isn’t enough. You need algorithms that scale efficiently, run fast, and use memory wisely. This is where algorithm analysis—especially concepts like asymptotic analysis, Big Omega, and algorithmic complexity—comes into play.
Understanding these concepts is vital for:
- Writing optimized code
- Acing technical interviews
- Competing in algorithm-based contests
- Building scalable, performant applications
This guide will help you master these foundational topics using practical explanations, theoretical insights, and real-world relevance.
⚙️ What is Algorithm Complexity?
📌 Definition
Algorithm complexity refers to the measure of resources (time and space) that an algorithm requires as a function of its input size. The two main aspects are:
- Time Complexity: How much time an algorithm takes to complete based on input size 'n'.
- Space Complexity: The total memory space the algorithm needs during execution.
These measurements help in selecting the right algorithm depending on the constraints and goals of your software system.
🧪 Real-World Relevance
Imagine searching a contact in your phone vs. scanning a paper address book. While both work, the efficiency (speed and effort) differs greatly. Similarly, some algorithms solve problems correctly but inefficiently—taking longer time or more memory than needed.
🧮 What is Asymptotic Analysis?
🧠 Definition
Asymptotic Analysis is a mathematical approach to evaluate an algorithm's efficiency, independent of hardware or implementation. It focuses on the algorithm’s behavior as the input size grows to infinity.
Asymptotic analysis helps compare algorithms objectively using standard notation.
🧭 Purpose
- Ignores machine-specific variables like processor speed, disk I/O, or compiler optimization.
- Focuses only on input size and operations performed.
This makes it a reliable way to compare algorithmic performance across platforms.
📊 Types of Asymptotic Notation
1. Big O Notation (O) – Worst Case
- Represents the upper bound.
- Measures the maximum time or resources an algorithm might take.
- Example: Bubble Sort → O(n²)
2. Big Omega Notation (Ω) – Best Case
- Represents the lower bound.
- Measures the minimum time an algorithm will take under ideal conditions.
- Example: Best case for Insertion Sort (already sorted input) → Ω(n)
3. Theta Notation (Θ) – Average/Tight Bound
- Represents both upper and lower bounds.
- Defines performance in average cases.
- Example: Merge Sort → Θ(n log n)
🔍 Deep Dive: What is Big Omega (Ω) Notation?
🧠 Big Omega Explained
Big Omega (Ω) is used to describe the best-case performance of an algorithm. It provides a lower bound, indicating the minimum amount of time or resources required for a particular input size.
🧪 Formal Definition
Let 'f(n)' be the time taken by an algorithm, and 'g(n)' be a function representing growth rate.
We say:
f(n) = Ω(g(n)) if there exist positive constants c and n₀ such that for all n ≥ n₀, f(n) ≥ c * g(n)
In simpler terms:
As the input grows, the algorithm will never run faster than 'g(n)' beyond a certain point.
🔄 Big Omega vs. Big O
-
Big O:
- Upper bound
- Describes the worst-case
- Used in industry & interviews
-
Big Omega:
- Lower bound
- Describes the best-case
- Less used but academically important
📌 Example: Linear Search
Best Case: If the element is the first in the list
- Ω(1): Constant time
Worst Case: If the element is at the end or not present
- O(n): Linear time
Thus, Linear Search is:
- Ω(1) best-case
- O(n) worst-case
✅ Why Big Omega Matters
- It helps estimate the best possible efficiency of an algorithm.
- Useful for understanding scenarios where input might be partially optimized.
- Important for theoretical analysis and academic evaluations.
🧮 Practical Example: Analyzing Time Complexity
Let’s consider a simple algorithm:
def sum_list(arr):
total = 0
for num in arr:
total += num
return total
Time Complexity:
- Each element is accessed once.
- T(n) = c × n → Linear
Thus:
- Best-case: Ω(n)
- Worst-case: O(n)
- Average-case: Θ(n)
Even in the best case, the entire array must be traversed. Hence, all notations match here.
🧬 Space Complexity
Definition
Space complexity represents the total memory an algorithm uses, including:
- Fixed Part: Constants, variables, program code.
- Variable Part: Data structures, recursion stack, input storage.
Example:
For a simple sum function using three variables (input array, loop variable, result):
- Fixed: O(1)
- Variable: Depends on array size → O(n)
So, total space complexity = O(n)
🧠 Apriori vs. Aposteriori Analysis
Apriori (Theoretical)
- Performed before implementation.
- Evaluates the algorithm abstractly.
- Uses mathematical models (like asymptotic notation).
Aposteriori (Empirical)
- Conducted after implementation.
- Performance is observed in real conditions.
- Includes benchmarking and profiling.
Why Apriori is Preferred
In professional development, software is used in diverse environments. Predicting efficiency via asymptotic analysis (apriori) ensures your code performs well regardless of the system it runs on.
🔢 Common Complexity Classes
-
Constant (O(1)):
- Same time regardless of input
- Example: Access array element
-
Logarithmic (O(log n)):
- Time grows slowly with input
- Example: Binary search
-
Linear (O(n)):
- Time grows linearly
- Example: Linear search
-
N log N (O(n log n)):
- Efficient sorts
- Example: Merge/Quick sort
-
Quadratic (O(n²)):
- Nested loops
- Example: Bubble sort
-
Cubic (O(n³)):
- Triple loops
- Example: Matrix multiplication
-
Exponential (O(2ⁿ)):
- Grows extremely fast
- Example: Recursive Fibonacci
-
Factorial (O(n!)):
- Worst case
- Example: Traveling salesman
💡 Key Takeaways
- Big Omega (Ω) helps analyze best-case performance.
- Asymptotic analysis is essential for platform-independent evaluation.
- Algorithm complexity includes both time and space factors.
- Use Big O, Ω, and Θ together for complete analysis.
- Prefer apriori analysis for scalable, general-purpose software.
❓ Frequently Asked Questions (FAQ)
1. What does Big Omega mean in algorithm analysis?
Big Omega (Ω) describes the minimum time or space an algorithm will need, indicating the best-case scenario for a given input size.
2. Is Big Omega used in real-world coding?
While not as common in interviews, Big Omega is essential in academic research and understanding full performance boundaries.
3. How is Big Omega different from Big O?
- Big O = Worst-case
- Big Omega = Best-case
Both are needed for full-spectrum analysis.
4. When should I use asymptotic analysis?
Use it when comparing algorithm efficiency regardless of hardware, especially before implementation (apriori).
5. What’s more important—time complexity or space complexity?
Both are critical. Time complexity is often prioritized, but space complexity becomes crucial in memory-constrained environments.
6. What tools can I use to analyze algorithms empirically?
- Python’s 'time' module
- Profilers like cProfile, gprof
- Benchmarking frameworks (e.g., Google Benchmark for C++)
🚀 Final Thoughts & Call to Action
Mastering Big Omega, asymptotic analysis, and algorithm complexity is crucial for every software engineer and computer science student. These foundational concepts empower you to:
- Choose better algorithms
- Write scalable code
- Pass coding interviews
- Build performance-driven systems
Start today by analyzing the time and space complexity of every algorithm you write. Not only will you improve as a developer—you’ll think like a computer scientist.
🔗 Recommended Resources
- MIT OpenCourseWare - Introduction to Algorithms
- Big-O Cheat Sheet
- GeeksforGeeks - Asymptotic Analysis
Related Articles
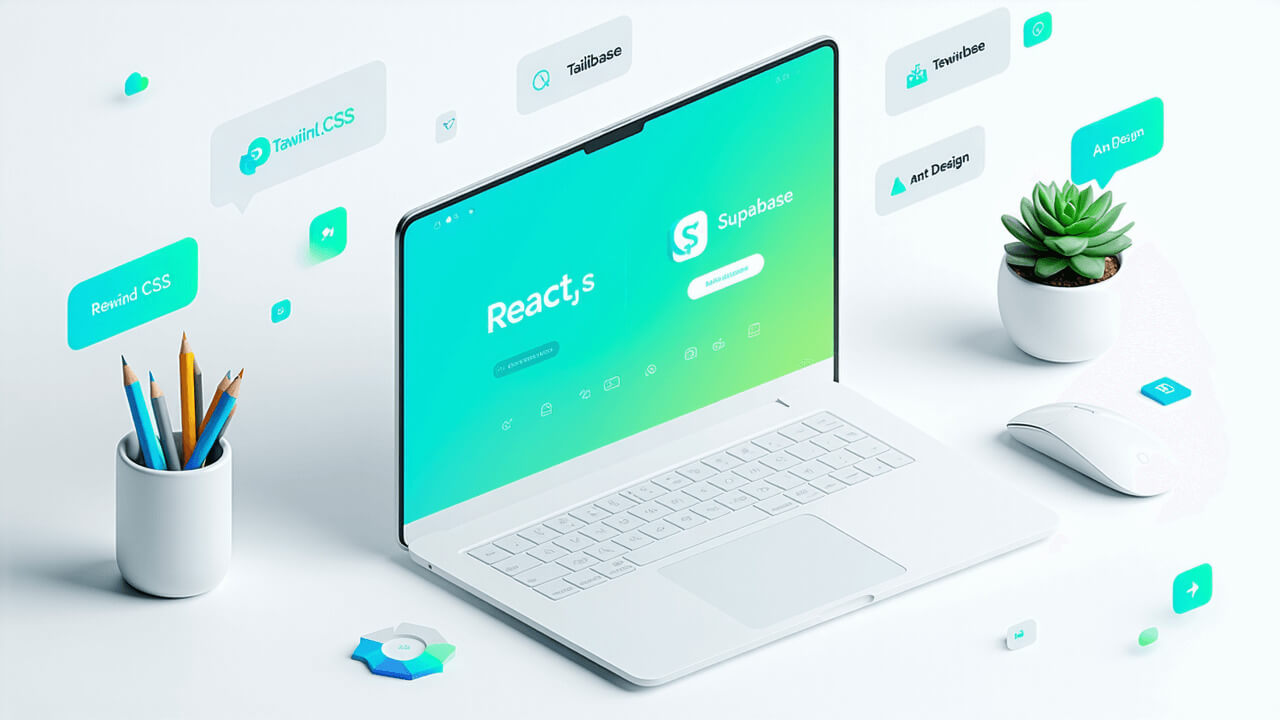
React.js and Supabase Masterclass – Build Full-Stack Apps from Scratch
Get started with full-stack development using React.js, Supabase, Tailwind CSS, and Ant Design. Learn authentication, file uploads, and deployment.
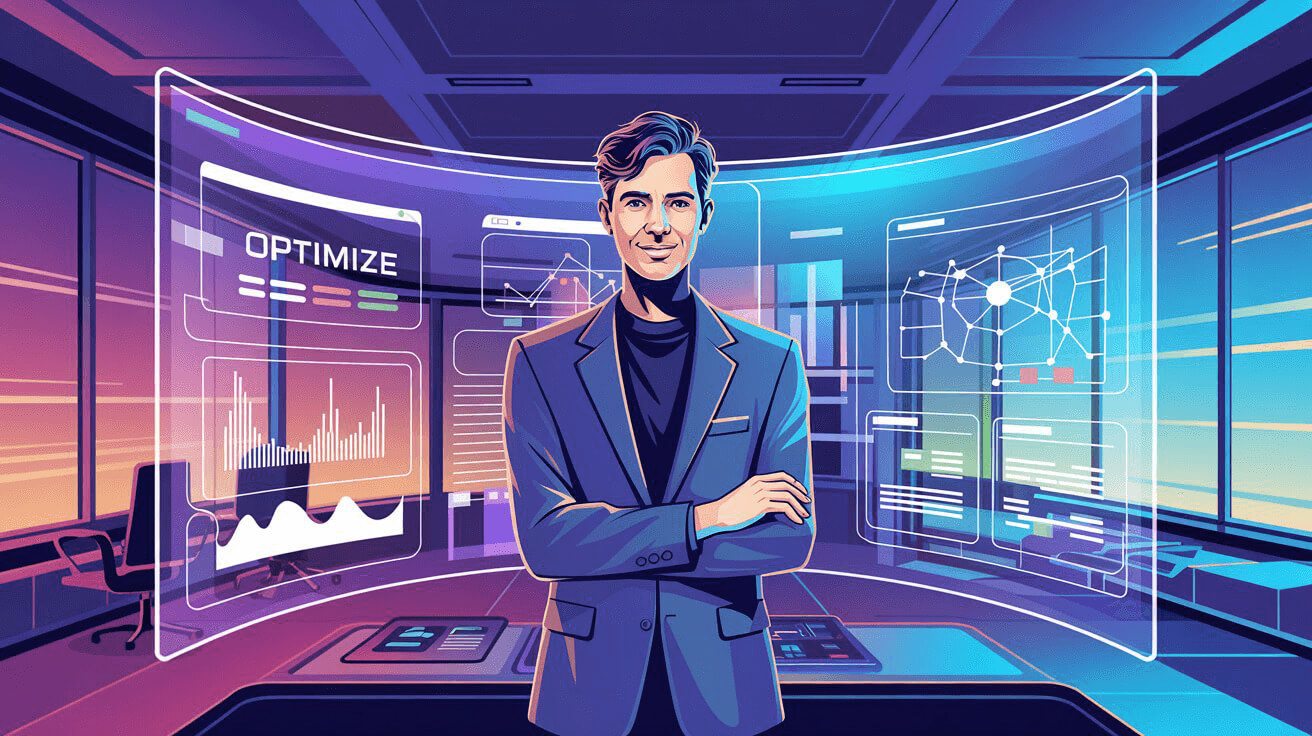
AI Product Management: Framework, Strategy & Career Guide
Master AI Product Management with actionable frameworks, real-world applications, and career growth strategies. Learn how to lead data-driven, value-focused AI products.